In this post, I will show you how to add Angular Material components in an Angular application.
Importing UI components directly in the app.module
increases the size of the module. The root module should be easy to maintain. Importing a button is not a great idea here.
For example, consider importing and configuring date picker in several feature modules. In this case, you can avoid duplication by having a single Material module.
Table of contents
Add Angular Material
First off, let’s add Angular Material to our project:
ng add @angular/material
Code language: Bash (bash)
This command installs Angular Material, the Component Dev Kit (CDK), Angular Animations.
It will ask these questions to setup Material according to your needs:
- Choose a prebuilt theme name, or “custom” for a custom theme
- Set up global Angular Material typography styles?
- Set up browser animations for Angular Material?
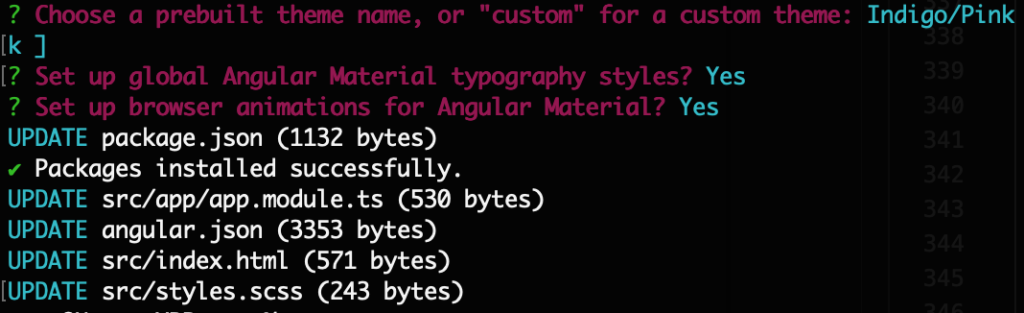
Generate feature module
Generate a module for keeping Material components:
ng generate module CustomMaterial
Code language: Bash (bash)
This will not only create a new folder called custom-material but also a module definition for your imports.
Next, open up the module definition and import a button:
//custom-material.module.ts
import { NgModule } from '@angular/core';
import { MatButtonModule } from '@angular/material/button';
@NgModule({
imports: [
MatButtonModule
],
exports: [
MatButtonModule
]
})
export class CustomMaterialModule {}
Code language: TypeScript (typescript)
Notice we need to export the button component, which makes it available to any module that imports the CustomMaterialModule
.
The idea here is that our custom material module will be responsible for any configuration, custom components etc. It should only export things that are needed by consumer modules.
At this point, you can import CustomMaterialModule
in any other module.
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
CustomMaterialModule,
BrowserAnimationsModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Code language: TypeScript (typescript)
We can now use the button in a component:
<button mat-raised-button> Hello </button>
Code language: HTML, XML (xml)
Going forward, you can just import the components from Angular Material into the CustomMaterialModule
and use them in your own components.
For example, if you need to display icons, you need to import and export MatIconModule
from the CustomMaterialModule
.
Custom components
You will reap the benefits of this approach, when the time comes to implement a custom feature into any of the Material components.
For example, if you implement a select all option for Select component, you can easily create a custom component inside the CustomMaterialModule
and export it for the whole app.
This way, you keep all related code in a single module, which makes it very easy to maintain the project.
Summary
To summarise, we looked at how you can add Angular Material as a feature module in an Angular app. Although this guide is focused on the Material library, the concept can be applied to any kind of UI framework. You could, for instance, import Kendo UI dependencies through a feature module.
Hi Umut, thanks for your write-up! It is really useful. I tried with the similar approach in my application and it works well when I use Material components inside app.components.ts but, if I use the material components in some other Custom components, it asks me to import Material Modules again in to that specific component. Doesn’t it work with importing Materials in app.module.ts itslef? Should I import the materials module in all the components wherever I use Ang. Materials?
Thanks for your comment! Yes, imports to root module will not be inherited in feature modules. You need to import material module in every feature module. Hope this helps, Umut